As per MDN The Promise
is an object that represents an asynchronous operation's eventual completion (or failure) and its resulting value.
Let’s break it down.
A promise is nothing but an object which consists of either the success or failure of an asynchronous task.
Javascript is a single-threaded language, and to handle async tasks promises came into the picture. They are commonly used when working with APIs or performing other time-consuming operations that need to be handled asynchronously.
A Promise
is in one of these states:
- Pending: The initial state. The promise is neither fulfilled nor rejected.
- Fulfilled: The operation was completed successfully, and the promise has a resulting value.
- Rejected: The operation failed, and the promise has a reason for the failure.
Here’s an example of how to create a promise in JavaScript:
const promise = new Promise((resolve, reject) => {// Perform some asynchronous operationconst result = doSomethingAsynchronous();// If the operation was successful, call the `resolve` function with the result// If the operation failed, call the `reject` function with the errorif (result) {resolve(result);} else {reject(new Error('Something went wrong!'));}});
In this example, we create a new promise using the Promise
constructor. The constructor takes a function as an argument, which is called the executor function. The executor function takes two parameters: resolve
and reject
.
Inside the executor function, we perform some asynchronous operations using doSomethingAsynchronous()
and then use an if
statement to check if it was successful. If the operation was successful, we call resolve
with the resulting value, and if it fails, we call reject
with an error object.
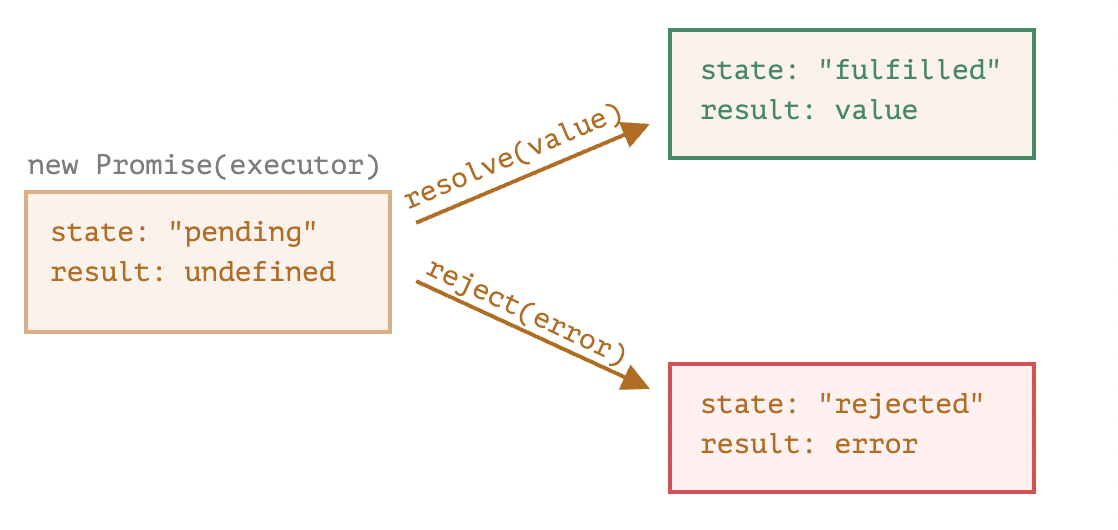
The promise
object returned by the new Promise
constructor has these internal properties:
state
— initially"pending"
, then changes to either"fulfilled"
whenresolve
is called or"rejected"
whenreject
is called.result
— initiallyundefined
, then changes tovalue
whenresolve(value)
is called orerror
whenreject(error)
is called.
Once the promise is created, we can handle its result using the then
and catch
methods. Here's an example:
In this example, we use thethen
method to handle the successful completion of the promise. If the promise is fulfilled, thethen
method is called with the resulting value as an argument. If the promise is rejected, we can use thecatch
method to handle the error.
As we know .then()
method takes up to two arguments; the first is a callback function for the fulfilled case of the promise, and the second is a callback function for the rejected case. Each .then()
returns a newly generated promise object, which can optionally be used for chaining. For example:
const myPromise = new Promise((resolve, reject) => {setTimeout(() => {resolve("Hello");}, 3000);});myPromise.then(handleFulfilledA).then(handleFulfilledB).then(handleFulfilledC).catch(handleRejectedAny);
Note: JavaScript is single-threaded by nature, so at a given instant, only one task will be executing, although control can shift between different promises, making execution of the promises appear concurrent.
Here’s another example of how to use promises in JavaScript with the fetch
API to make an HTTP request:
fetch('https://api.example.com/data').then(response => {if (!response.ok) {throw new Error('Network response was not ok');}return response.json();}).then(data => {console.log('Data:', data);}).catch(error => {console.error('Error:', error);});
In this example, we use the fetch
function to make an HTTP request to an API endpoint. We then use the then
method to handle the response from the API. If the response is not ok
, we throw an error using the throw
keyword. If the response is ok
, we use the json
method to extract the JSON data from the response.
Once we have the data, we use another then
method to handle it and log it to the console. If an error occurs at any point in the process, we use the catch
method to handle it.
In conclusion, promises in JavaScript are a powerful tool for working with asynchronous operations. They allow us to handle the results of these operations in a clean and concise way, using the then
and catch
methods. By understanding how promises work and how to use them, we can write more efficient and robust code that is able to handle a wide range of asynchronous scenarios.
In case I have missed something or you have some advice or suggestions do let me know in the comment section.
You can also reach out to me
https://www.linkedin.com/in/akhatun/
https://www.instagram.com/readwithamnah/
https://readwithamnah.blogspot.com/
Happy coding! ✌️
Comments
Post a Comment